If you’re new to the world of electronics and eager to explore wireless communication, interfacing XBee S2 (ZigBee) modules with an Arduino UNO is a great starting point. This guide will walk you through the process step-by-step, making it easy for beginners to understand and implement. By the end of this tutorial, you’ll be able to set up and use XBee modules for wireless data transmission with your Arduino projects. Let’s dive in and start connecting!
Overview of XBee S2
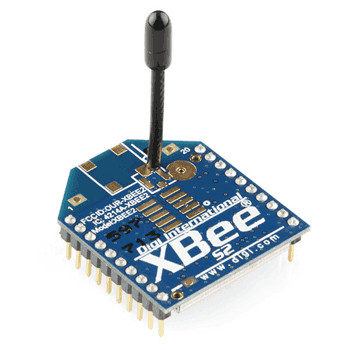
XBee (ZigBee) radios are based on the IEEE 802.15.4 standard, which defines the operation of low-rate wireless personal area networks (LR-WPANs). They are designed for communication methods such as point-to-point and star networks over the air.
ZigBee is an IEEE 802.15.4-based specification for high-level communication protocols used to create personal area networks with low-power digital radios.
Key features of XBee radio devices include:
- Operating on the 2.4 GHz unlicensed radio band.
- Low data rate of approximately 250 Kbps.
- Low power consumption options (e.g., 1mW, 6mW, 250mW).
- Suitable for wireless communication over short distances (e.g., 90m, 750m, 1 mile).
Due to these features, XBee radios are commonly used in applications such as home automation, wireless sensor networks, industrial control, medical data collection, and building automation.
For more information on how XBee modules work, refer to the XBee Module topic in the sensors and modules section.
Connection Diagram of Xbee S2 Module with Arduino
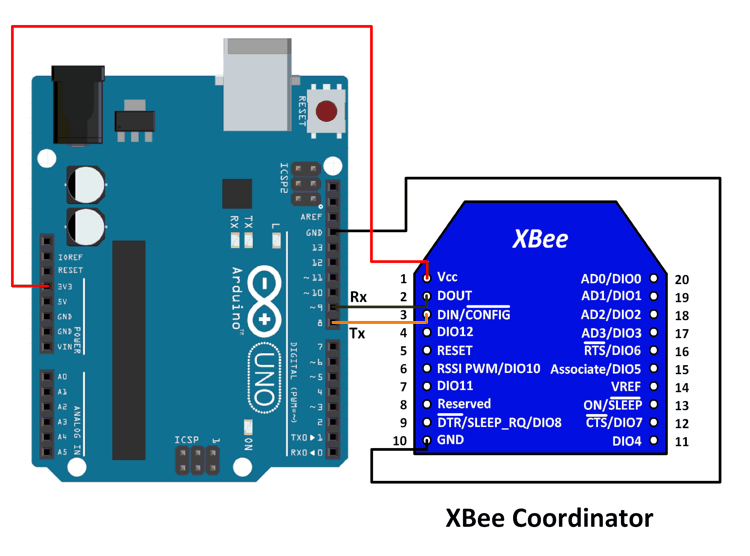
Parts Required
Component Name | Buy Now |
Arduino Uno REV3 | Amazon |
Xbee 3 Module – RP-SMA Antenna Connector | Amazon |
XBee Module Series Upgrade S2 S2C Zigbee Module Wireless | Amazon |
Connection Diagram of XBee Router (At End device)
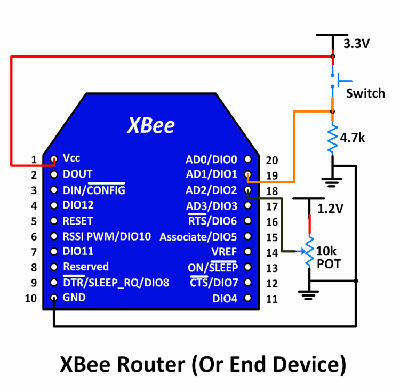
XBee S2 Example Using Arduino UNO
In this example, we connect an XBee S2 module to an Arduino UNO. The XBee is set up as a Coordinator in API mode, with the API enable setting set to 1 (you can also set it to 2, which uses escape characters along with the data).
We use SoftwareSerial on the Arduino for communication with the XBee, while the Serial port on the Arduino is used to display received data on the serial monitor.
Another XBee device is configured as a Router in API mode, also with the API enable setting set to 1 (or 2 for escape characters). Both XBee devices should have the same API enable setting. Alternatively, you can configure this device as an End Device in API mode.
A switch is connected to pin DIO1 (pin 19 on the module) of the Router (or End Device) XBee module, and this pin is configured as a Digital Input.
A potentiometer is connected to 1.2V and ground on its fixed terminals, with its variable terminal connected to pin AD2 (pin 18 on the module) of the Router (or End Device) XBee module, configured as an Analog Input.
The IO Sampling (IR) rate can be set according to your application needs, such as 100 msec.
All configurations and settings are done using the X-CTU software from Digi International.
If you’re unsure how to configure XBee modules using X-CTU, refer to the XBee Configuration sub-topic under XBee Module in the sensors and modules section.
The Router (or End Device) XBee module periodically sends IO samples from the potentiometer and switch based on the IR setting. This data is received by the Coordinator, and the sketch uploaded to the Arduino parses the received data to extract the IO samples information.
This information is then displayed on the serial monitor to verify proper operation.
We will use Andrew Rapp’s XBee library for Arduino, which you can download from GitHub.
Extract the library and add it to the libraries folder in the Arduino IDE.
For instructions on adding a custom library to the Arduino IDE and using examples from it, refer to Adding Library to Arduino IDE in the Basics section.
In this example, we’ve modified the author’s example sketch for receiving IO samples for S2 modules. The modifications primarily swap the Serial ports used for communication and debugging to avoid using an additional device for debugging.
XBee S2 (ZigBee) Code for Arduino UNO
#include <XBee.h> #include <SoftwareSerial.h> #define ssRX 9 /* Rx pin for software serial */ #define ssTX 8 /* Tx pin for software serial */ /* Create object named xbee_ss of the class SoftwareSerial */ SoftwareSerial xbee_ss(ssRX, ssTX); /* Define pins for software serial instance named xbee-ss(any name of your choice) to be connected to xbee */ /* ssTx of Arduino connected to Din (pin 3 of xbee) */ /* ssRx of Arduino connected to Dout (pin 2 of xbee) */ XBee xbee = XBee(); /* Create an object named xbee(any name of your choice) of the class XBee */ ZBRxIoSampleResponse ioSamples = ZBRxIoSampleResponse(); /* Create an object named ioSamples(any name of your choice) of the class ZBRxIoSampleResponse */ void setup() { Serial.begin(9600); /* Define baud rate for serial communication */ xbee_ss.begin(9600); /* Define baud rate for software serial communication */ xbee.setSerial(xbee_ss); /* Define serial communication to be used for communication with xbee */ /* In this case, software serial is used. You could use hardware serial as well by writing "Serial" in place of "xbee_ss" */ /* For UNO, software serialis required so that we can use hardware serial for debugging and verification */ /* If using a board like Mega, you can use Serial, Serial1, etc. for the same, and there will be no need for software serial */ } void loop() { xbee.readPacket(); /* Read until a packet is received or an error occurs */ if(xbee.getResponse().isAvailable()) /* True if response has been successfully parsed and is complete */ { if(xbee.getResponse().getApiId()==ZB_IO_SAMPLE_RESPONSE) /* If response is of IO_Sample_response type */ { xbee.getResponse().getZBRxIoSampleResponse(ioSamples); /* Get the IO Sample Response */ Serial.print("Received I/O Sample from: "); Serial.print(ioSamples.getRemoteAddress64().getMsb(), HEX); /* DH(in HEX format) of the sending device */ Serial.print(ioSamples.getRemoteAddress64().getLsb(), HEX); /* DL(in HEX format) of the sending device */ Serial.println(""); if (ioSamples.containsAnalog()) { /* If Analog samples present in the response */ Serial.println("Sample contains analog data"); } if (ioSamples.containsDigital()) { /* If Digital samples present in the response */ Serial.println("Sample contains digtal data"); }
Code Explanation
This code uses an XBee module to communicate with an Arduino UNO. The communication between the XBee and Arduino is established using the SoftwareSerial library, which allows serial communication on other digital pins of the Arduino.
Here’s a detailed explanation of the code:
Libraries and Definitions
#include <XBee.h> #include <SoftwareSerial.h> #define ssRX 9 /* Rx pin for software serial */ #define ssTX 8 /* Tx pin for software serial */
- The
XBee
library provides functions to interact with the XBee module. - The
SoftwareSerial
library allows serial communication on digital pins other than the default RX and TX pins (0 and 1). ssRX
andssTX
define the pins for software serial communication. These pins will be used to communicate with the XBee module.
Creating Objects
SoftwareSerial xbee_ss(ssRX, ssTX); /* Define pins for software serial instance named xbee_ss to be connected to XBee */ /* ssTX of Arduino connected to Din (pin 3 of XBee) */ /* ssRX of Arduino connected to Dout (pin 2 of XBee) */ XBee xbee = XBee(); /* Create an object named xbee of the class XBee */ ZBRxIoSampleResponse ioSamples = ZBRxIoSampleResponse(); /* Create an object named ioSamples of the class ZBRxIoSampleResponse */
xbee_ss
is an instance of theSoftwareSerial
class, configured to use pins 8 (TX) and 9 (RX) for serial communication with the XBee.xbee
is an instance of theXBee
class, used to manage communication with the XBee module.ioSamples
is an instance of theZBRxIoSampleResponse
class, used to handle I/O sample responses from the XBee module.
Setup Function
void setup() { Serial.begin(9600); /* Define baud rate for serial communication */ xbee_ss.begin(9600); /* Define baud rate for software serial communication */ xbee.setSerial(xbee_ss); /* Define serial communication to be used for communication with XBee */ /* In this case, software serial is used. You could use hardware serial as well by writing "Serial" in place of "xbee_ss" */ /* For UNO, software serial is required so that we can use hardware serial for debugging and verification */ /* If using a board like Mega, you can use Serial, Serial1, etc. for the same, and there will be no need for software serial */ }
Serial.begin(9600)
initializes the hardware serial port for debugging at a baud rate of 9600.xbee_ss.begin(9600)
initializes the software serial port at a baud rate of 9600.xbee.setSerial(xbee_ss)
sets the software serial instance as the communication port for the XBee object.
Loop Function
void loop() { xbee.readPacket(); /* Read until a packet is received or an error occurs */ if(xbee.getResponse().isAvailable()) /* True if response has been successfully parsed and is complete */ { if(xbee.getResponse().getApiId()==ZB_IO_SAMPLE_RESPONSE) /* If response is of IO_Sample_response type */ { xbee.getResponse().getZBRxIoSampleResponse(ioSamples); /* Get the IO Sample Response */ Serial.print("Received I/O Sample from: "); Serial.print(ioSamples.getRemoteAddress64().getMsb(), HEX); /* DH(in HEX format) of the sending device */ Serial.print(ioSamples.getRemoteAddress64().getLsb(), HEX); /* DL(in HEX format) of the sending device */ Serial.println(""); if (ioSamples.containsAnalog()) { /* If Analog samples present in the response */ Serial.println("Sample contains analog data"); } if (ioSamples.containsDigital()) { /* If Digital samples present in the response */ Serial.println("Sample contains digital data"); } } } }
xbee.readPacket()
reads packets from the XBee module until a packet is received or an error occurs.xbee.getResponse().isAvailable()
checks if a response has been successfully parsed and is complete.xbee.getResponse().getApiId()==ZB_IO_SAMPLE_RESPONSE
checks if the response is of the IO Sample Response type.xbee.getResponse().getZBRxIoSampleResponse(ioSamples)
retrieves the IO Sample Response and stores it inioSamples
.- The address of the sending device is printed in HEX format.
ioSamples.containsAnalog()
checks if the response contains analog data and prints a message if true.ioSamples.containsDigital()
checks if the response contains digital data and prints a message if true.
This code sets up the Arduino to receive and display I/O samples from an XBee module, facilitating the monitoring of digital and analog inputs remotely.