If you’re interested in maintaining a record of your greenhouse’s climate, constructing a humidor control system, or monitoring temperature and humidity data for a weather station project, the SHT31 Temperature & Humidity Sensor could be the perfect solution for you!
The SHT31 sensor comes pre-calibrated from the factory and doesn’t need any additional components to function. With minimal connections and a bit of Arduino programming, you can begin measuring relative humidity and temperature immediately.
Parts Required
Hardware Overview
Featuring a digital temperature and humidity sensor from Sensirion – the SHT31 – this module offers a low-cost, user-friendly, and highly accurate solution.
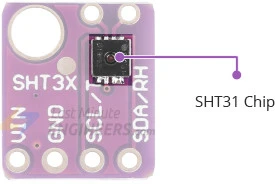
Its compact size makes it versatile for various applications, including thermostats, humidistats, indoor weather stations, and similar devices, facilitating humidity and/or temperature monitoring or control.
The SHT31 sensor provides precise humidity readings across the entire 0 to 100% RH range, with an average accuracy of ±2% within the 20% to 80% RH range (offering a resolution of 0.01% RH).
Operating within a temperature range of -40 to 125°C, the SHT31 maintains a typical accuracy of ±0.3°C at 25°C (with a resolution of 0.015°C).
The SHT31 sensor features a small window exposing the polymer sensing film responsible for temperature and humidity measurement. It is important to prevent liquids, dust, or other contaminants from contacting it, as this could affect the sensor’s accuracy.
Power Requirement
The sensor operates within a voltage range of 2.4V to 5.5V, making it compatible with both 3V and 5V systems. This versatility ensures seamless integration with your preferred microcontroller, whether it operates at 3.3V or 5V.
During measurements, the SHT31 consumes less than 0.8mA of power, while in single-shot mode (not actively measuring), it draws less than 0.2µA. This low power consumption makes it ideal for use in battery-powered devices such as handheld devices, wearables, or smartwatches.
I2C Interface
The SHT31 utilizes the I2C communication protocol, utilizing the standard data and clock wires available on most microcontrollers. It can coexist with other I2C sensors on the same bus, provided there are no address conflicts.
It offers two distinct I2C addresses: 0x44Hex and 0x45Hex, allowing for the simultaneous use of two SHT31 modules on the same bus or avoiding address clashes with other devices.
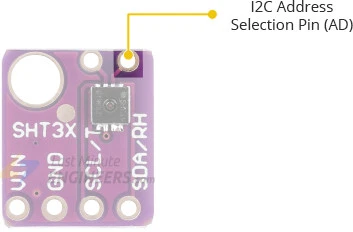
The module’s I2C address is determined by the state of the AD pin, which features a built-in pull-down resistor. Leaving the AD pin unconnected defaults the I2C address to 0x44Hex, while connecting it to a high-voltage signal sets the address to 0x45Hex.
Alert Mode
Equipped with an alert (AL) output pin, the SHT31 can signal when environmental conditions (humidity and/or temperature) surpass user-defined thresholds. This feature enables interrupt-driven measurements, freeing the host microcontroller to execute other tasks while the sensor collects data.
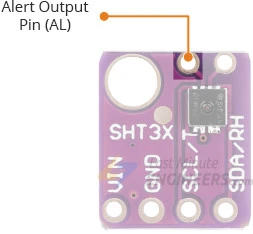
When the humidity and/or temperature exceeds the upper limit, the Alert pin goes HIGH and remains so until the temperature drops below the clear threshold. Similarly, if the humidity and/or temperature falls below the lower limit, the alert pin goes HIGH until the temperature rises above the clear threshold.
Refer to a separate application note for further details and illustrated examples of the Alert Mode.
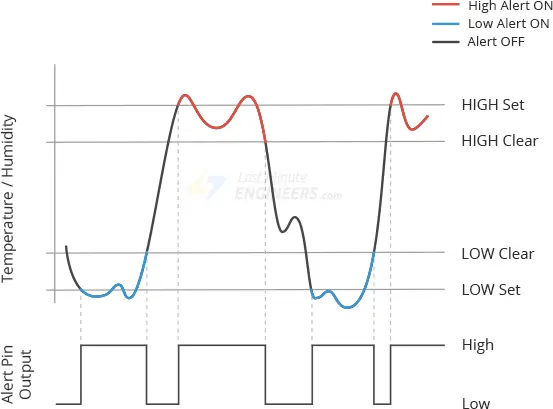
Technical Specifications
Here are the complete specifications:
Power supply | 2.4V to 5.5V |
Current draw | ~0.8mA (during measurements) |
~0.2µA (during single shot mode) | |
Humidity Range | 0 to 100 %RH |
Humidity Accuracy | ±2% over the range of 20% to 80% RH |
Temperature Range | -40˚C to +125˚C |
Temperature Accuracy | ±0.3˚C at 25°C |
For more details, please refer below datasheet.
SHT31 Module Pinout
Let’s examine the pinout of the SHT31 module.
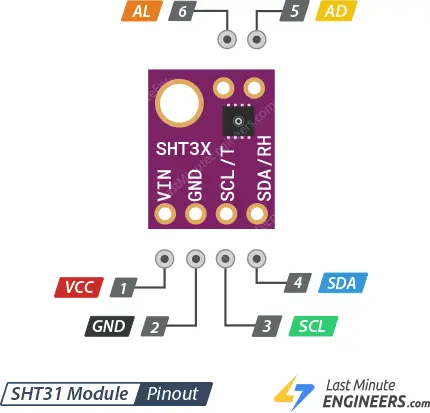
- VCC is the power pin. As the sensor operates within a range of 2.4-5.5VDC, supply it with the same voltage as the logic level of your microcontroller. For instance, if you’re using a 5V microcontroller like Arduino, provide 5V power.
- GND serves as the common ground for both power and logic.
- SCL is the I2C clock pin, to be connected to your microcontroller’s I2C clock line.
- SDA is the I2C data pin, to be connected to your microcontroller’s I2C data line.
- The AD pin determines the I2C address of the module. Leaving the AD pin unconnected sets the default I2C address to 0x44Hex, while connecting it to a high-voltage signal sets the address to 0x45Hex.
- The AL pin triggers when environmental conditions (humidity and/or temperature) surpass user-defined thresholds.
Wiring up a SHT31 Module to an Arduino
Connecting the SHT31 sensor is straightforward!
Only four pins need to be connected to begin using the sensor: one for VCC, one for GND, and two for data lines for I2C communication.
Link the SCL pin to the I2C clock pin and the SDA pin to the I2C data pin on your Arduino. It’s important to note that different Arduino boards have different I2C pins, which should be connected accordingly. On Arduino boards with the R3 layout, the SDA (data line) and SCL (clock line) pins are located near the AREF pin and are labeled A4 (SDA) and A5 (SCL).
The following illustration depicts the wiring setup.
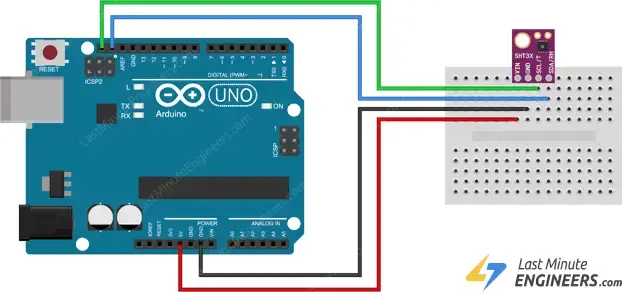
Library Installation
To set up your sensor, you’ll need to install the Adafruit SHT31 library, accessible through the Arduino library manager.
Begin by navigating to Sketch > Include Library > Manage Libraries… Allow the Library Manager to download the libraries index and update the list of installed libraries.
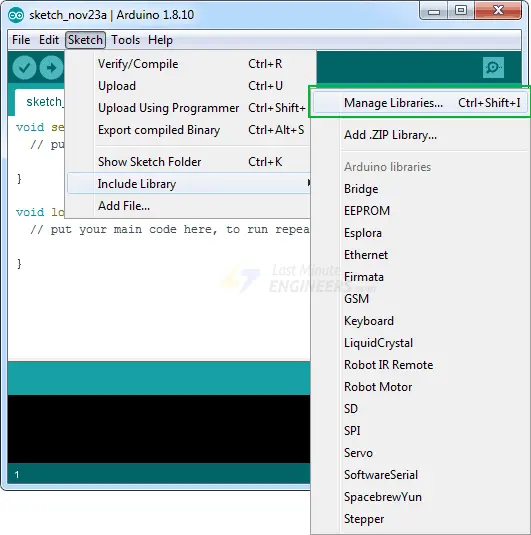
Filter your search by typing ‘SHT31’ and proceed to install the library.
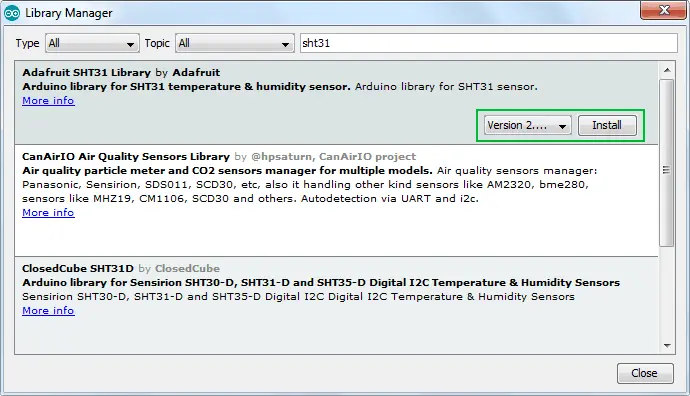
Additionally, the Adafruit_SHT31 library utilizes the Adafruit Bus IO helper library internally to simplify I2C & SPI transactions and registers. Therefore, search for ‘adafruit bus’ in the library manager and install it alongside the SHT31 library.

Arduino Code – Reading Temperature and Humidity
Below is a basic Arduino sketch. Go ahead and upload it to your Arduino. You will see the current temperature and humidity in your room!
#include <Arduino.h> #include <Wire.h> #include "Adafruit_SHT31.h" Adafruit_SHT31 sht31 = Adafruit_SHT31(); void setup() { Serial.begin(9600); if (! sht31.begin(0x44)) { // Set to 0x45 for alternate I2C address Serial.println("Couldn't find SHT31"); while (1) delay(1); } } void loop() { float t = sht31.readTemperature(); float h = sht31.readHumidity(); if (! isnan(t)) { // check if 'is not a number' Serial.print("Temp *C = "); Serial.print(t); Serial.print("\t\t"); } else { Serial.println("Failed to read temperature"); } if (! isnan(h)) { // check if 'is not a number' Serial.print("Hum. % = "); Serial.println(h); } else { Serial.println("Failed to read humidity"); } delay(1000); }
Once your code is uploaded, open the serial terminal at 9600bps. You should see something like the output below. Try breathing on the sensor to see both humidity and temperature values change!
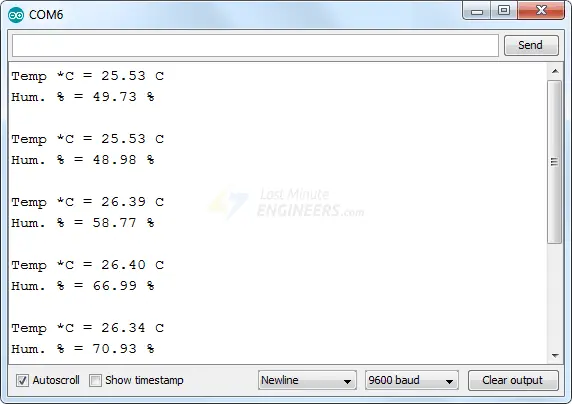
Code Explanation:
The code is straightforward. It begins by including the Arduino.h
, Wire.h
, and Adafruit_SHT31.h
libraries. Then, an Adafruit_SHT31 object is created in the global scope.
#include <Arduino.h> #include <Wire.h> #include "Adafruit_SHT31.h" Adafruit_SHT31 sht31 = Adafruit_SHT31();
In the setup function, serial communication with the PC is initialized, and the begin()
function is called.
The begin(<address>)
function initializes the sensor, where <address>
is the I2C address of the sensor. By default, it’s set to 0x44Hex, but you can adjust the sensor for 0x45Hex and then pass that value in. This function returns True if the sensor was found and responded correctly, and False if it was not found.
void setup() { Serial.begin(9600); if (! sht31.begin(0x44)) { // Set to 0x45 for alternate I2C address Serial.println("Couldn't find SHT31"); while (1) delay(1); } }
Once initialized, you can access the object’s (sht31) methods using the dot operator.
- sht31.readTemperature() returns a floating-point temperature reading in °C. You can convert it to Fahrenheit by multiplying by 1.8 and adding 32.
- sht31.readHumidity() returns the humidity reading as a floating-point value between 0 and 100 (representing % humidity).
void loop() { float t = sht31.readTemperature(); float h = sht31.readHumidity(); if (! isnan(t)) { // check if 'is not a number' Serial.print("Temp *C = "); Serial.print(t); Serial.print("\t\t"); } else { Serial.println("Failed to read temperature"); } if (! isnan(h)) { // check if 'is not a number' Serial.print("Hum. % = "); Serial.println(h); } else { Serial.println("Failed to read humidity"); } delay(1000); }
The loop continuously reads temperature and humidity values from the sensor and prints them to the serial monitor. If a reading fails, an error message is printed. The delay(1000) function introduces a 1-second delay between readings.
Related article
- Step-by-Step: Interfacing Multiple DS18B20 Sensors with Arduino
- MLX90614 Integration Guide for Arduino Temperature Sensing
- Arduino DHT11 Sensor Tutorial: How to Interface and Read Data
- Arduino DHT11 and DHT22 Sensor Interface: Step-by-Step Guide
- MAX6675 Thermocouple Module: Seamless Integration with Arduino
- Arduino-Compatible HTU21D Sensor: Monitor Temp & Humidity Easily